by David Loo | Mar 19, 2013 | Sharepoint Development
In this blog post I will explain and demonstrate how to Add, Update and Delete a SharePoint List Item using the server side object model.
Adding a New Item
In the code sample below I obtain the current context and the web and get the list I would like to add the new item to. After I have assigned values to my fields I call an update method from the item.
SPWeb spWeb = SPContext.Current.Web;
SPList spList = spWeb.Lists["MyList"];
SPListItem item = spList.Items.Add();
item["Title"] = "Hello, world!";
item.Update();
Update an Existing Item
Updating an existing item from a SharePoint List is very similar to adding a new item, but the only difference is instead of calling Add() from the SPListItemCollection, I now call the GetItemById(int) from the SPList object. There are other ways of getting an item from a list and another one is GetItemByTitle.
SPWeb spWeb = SPContext.Current.Web;
SPList spList = spWeb.Lists["MyList"];
SPListItem item = spList.GetItemById(1);
item["Title"] = "Hello, world!";
item.Update();
Deleting an Existing Item
Deleting an existing item from a SharePoint List all I need to do is get the item I want from this list and then call the Delete method from the item object. That’s how easy it is.
SPWeb spWeb = SPContext.Current.Web;
SPList spList = spWeb.Lists["MyList"];
SPListItem item = spList.GetItemById(1);
item.Delete();
I hope the information I have provided in this post is useful to you.
by David Loo | Sep 23, 2012 | Sharepoint Development
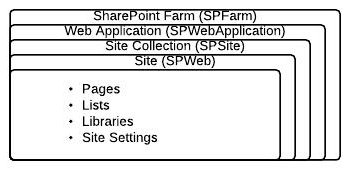
I writing this post to let others understand the basics of the SharePoint hierarchy and the Object Model. I know as a developer it can get confusing with the terminologies. This post I would like to address the main areas of the hierarchy and explain their purposes:
Farm:
The farm is a collection of
- front end web servers – these are the web site or also known as web applications
- application servers – these are the servers that provide the back end services such as searching, office services and etc.
- databases servers – these are the servers that stores the configuration content of the whole farm.
In the SharePoint Object Model the SPFarm class is the first tier of the hierarchy, this class consist of two properties:
- Servers – this property gets all the servers in the farm in a collection of SPServer objects.
- Services – this property gets all the services in the farm in a collection of SPService objects. (more…)
by David Loo | Sep 2, 2012 | Sharepoint Development
I been working on a project recently and one of the pages required a list view from another site from the same site collection. So this is what I had to do to make it work.
var site = SPContext.Current.Site;
var spList = site.AllWeb["yourwebsite"].Lists["listname"];
var xsltLvWp = new XsltListViewWebPart(){
Title = "My Cross Site List View",
WebId = spList.ParentWeb.ID,
ListName = spList.ID.ToString("B").ToUpper(),
ListId = spList.ID,
ViewGuid = spList.Views["View Name"].ID.ToString("B").ToUpper()
}
by David Loo | Jun 25, 2012 | Sharepoint Development
To bind a SPList to a binding control list for example the DetailsView data control you will need to provide a DataSource to it. The problem is SPList doesn’t implment the IDataSource interface, but I have discovered that there is a SPDataSource which allows you to pass a SPList object as a parameter in the constructor. Below is a code
var spList = SPContext.Current.Web.Lists["List Name"];
var ds = new SPDataSource(spList);
DetailsView1.DataSource = ds;
DetailsView1.DataBind();